To get started let’s update our computers and install a python framework known as Flask that is going to get us mapping quickly! Flask is one example of a Model View Controller or MVC for short. The concept here is that we have separate components to make updates easy. For example in the case of a Social Network our model would define what a post is, what types of content it contains, etc. The controller decides hows to handle that data, IE put the data in a database, put images on a content delivery network, send notifications to subscribers etc. And a view is how that is displayed, the website is a view, the mobile website, and mobile apps again separate views.
When starting out there will feel like a lot of repeating yourself and you define concepts in each of the components, but there are dividends that will pay off on longer-lived projects. The advantage of this system is that if we want to change our database or the CDN provider we can change the controller but it will in no way affect our published mobile apps. Additionally, because all of our views interface with the model we get a consistent experience of data, but the freedom to display based on device.
sudo apt update && sudo apt upgrade sudo apt install python3-venv python3-flask
Next, we are going to cd into our git folder, and make a directory called flask-map. Once cd’d into this new folder run this command to make a virtual environment. A virtual environment is essentially a set of packages that will be used for this task and allows us to keep our packages at different update levels than the rest of the system.
python -m venv map-env
If evey thing worked you should now have a folder structure that looks something along the lines of \home\username\GEOG413-username\flask-map\map-env.
Now we need to activate this version of python, use the source command and point it to map-env/bin/activate. If it works your terminal should have (map-env) at the start of each line.

And finally lets install the python packages we will be using
pip install numpy python-dateutil pytz geopy pandas flask flask_sqlalchemy geoalchemy2 psycopg2-binary
make a file called map.py with the following contents
from flask import Flask app = Flask(__name__) @app.route('/') def hello(): return 'Hello, World!'
Then run the following commands to run your new flask app in a development mode.
export FLASK_APP=map export FLASK_ENV=development flask run
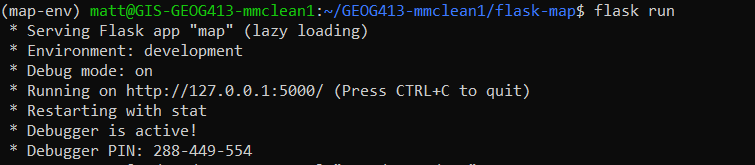
You should now be able to visit http://127.0.0.1:5000/ on your virtual machine and see a message that says Hello World!.
But we are GIS People we don’t just want to say hello we want maps!
Change your map.py to look like this:
from flask import Flask from flask import render_template app = Flask(__name__) @app.route('/') def hello(): return 'Hello, World!' @app.route('/map') def mapp(): return render_template("map.html")
And add a folder called ‘templats’ (The name matters as it is a keyword), inside of your templates folder a file called map.html. What we are doing is displaying this website when /map is added to the end of the url.